Building Secure Forms in Next.js: A Comprehensive Guide
Learn how to build a secure form Using Nextjs and React-hook-form and yup.
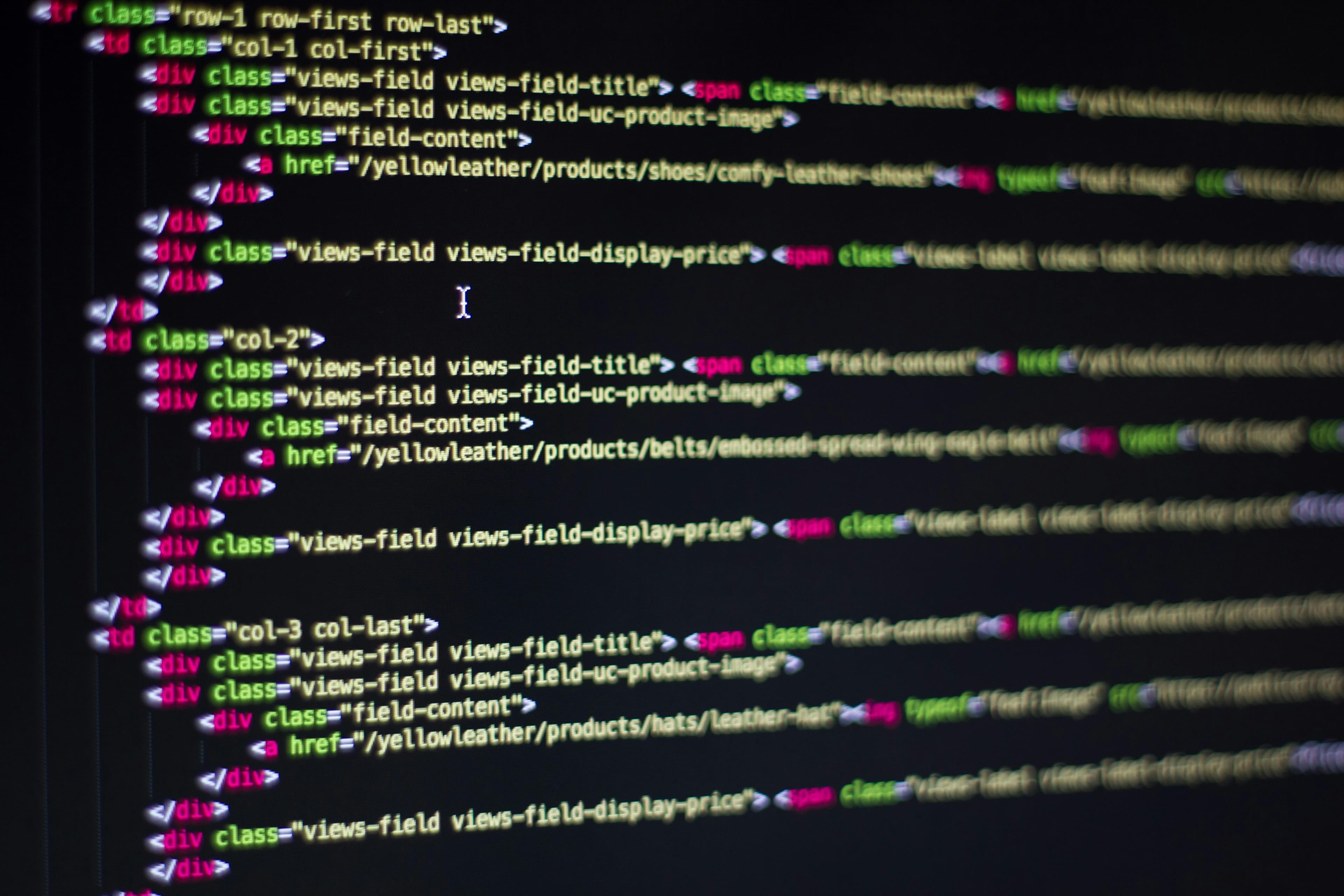
In this guide, we will explore how to build secure, user-friendly forms in Next.js using a powerful combination of libraries:
-
React-Hook-Form - Simplifies form state management and user interactions.
-
Yup - Provides robust schema validation.
-
Tailwind CSS - Efficiently styles our forms.
Why Secure Forms Matter
Insecure forms can be exploited through attacks like SQL injection and cross-site scripting (XSS), compromising your application's security and potentially stealing sensitive data. Proper validation ensures data integrity and enhances user experience.
Step-by-Step Guide to Creating Secure Forms
1. Project Setup
First, create a new Next.js project and install the required dependencies:
npx create-next-app@latest my-secure-form
2. Form Component (Form.js)
Create a form component using React-Hook-Form, Yup, and Tailwind CSS:
import { useForm } from 'react-hook-form';
import { yupResolver } from '@hookform/resolvers/yup';
import * as Yup from 'yup';
const validationSchema = Yup.object().shape({
name: Yup.string().required('Name is required'),
email: Yup.string().email('Email is invalid').required('Email is required'),
});
const Form = () => {
const { register, handleSubmit, formState: { errors } } = useForm({
resolver: yupResolver(validationSchema),
});
const onSubmit = data => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)} className="bg-white shadow-md rounded px-8 pt-6 pb-8 mb-4">
<div className="mb-4">
<label htmlFor="name" className="block text-gray-700 text-sm font-bold mb-2">Name</label>
<input
{...register('name')}
type="text"
id="name"
className="shadow appearance-none border rounded w-full py-2 px-3 text-gray-700 leading-tight focus:outline-none focus:shadow-outline"
placeholder="Enter your name"
/>
{errors.name && <span className="text-red-500 text-sm">{errors.name.message}</span>}
</div>
<div className="mb-4">
<label htmlFor="email" className="block text-gray-700 text-sm font-bold mb-2">Email</label>
<input
{...register('email')}
type="email"
id="email"
className="shadow appearance-none border rounded w-full py-2 px-3 text-gray-700 leading-tight focus:outline-none focus:shadow-outline"
placeholder="Enter your email"
/>
{errors.email && <span className="text-red-500 text-sm">{errors.email.message}</span>}
</div>
<button type="submit" className="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded focus:outline-none focus:shadow-outline">
Submit
</button>
{errors.submit && <span className="text-red-500 text-sm">Form submission failed. Please try again.</span>}
</form>
);
};
export default Form;
3. Integrate Form Component in a Page
Create a page to display the form:
import Form from '../components/Form';
const MyPage = () => {
return (
<div className="container mx-auto px-4 py-8">
<h1 className="mb-6 text-3xl font-bold">Contact Us</h1>
<Form />
</div>
);
};
export default MyPage;
Key Considerations
Server-Side Validation
Always validate user input on the server-side to prevent malicious attacks. Sanitize and escape user input before processing it. Even with client-side validation, server-side validation is crucial for robust security.
API Endpoints
If you're handling form submissions on the server, create a secure API endpoint in a Next.js API route to receive and process form data.
Conclusion
By combining React-Hook-Form, Yup, and Tailwind CSS, you can create secure and well-validated forms in your Next.js applications. This approach improves data integrity, user experience, and protects your application from security vulnerabilities.