A Deep Dive into Event Emitters in Node.js
Learn how to leverage your skills and capitalize on various platforms to boost your income and thrive in the digital landscape.
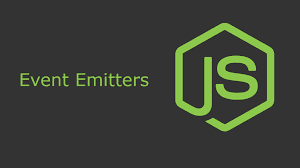
In Node.js, events play a central role in handling asynchronous operations, which are crucial for building efficient and scalable applications. At the core of event-driven architectures is the EventEmitter class, which enables objects to emit events and allows other parts of your code to listen to them.
In this article, we’ll explore the EventEmmiter in-depth, understand its internals, and demonstrate how it works through examples. By the end, you’ll have a strong understanding of how to use events in your Nodejs applications.
What is an EventEmitter?
In Node.js, much of the asynchronous functionality such as reading files, making HTTP requests, or interacting with databases is built using an event-driven model. The EventEmitter
class is part of Node.js’s events
module, which allows objects to communicate with one another by emitting and listening to events. You can define custom events and bind listeners (handlers) to them. When an event is emitted, all the bound listeners are invoked.
EventEmitter Structure
The EventEmitter
is a class that comes from Node.js's built-in events
module. It acts as a hub where events are emitted, and listeners are triggered based on those events. The typical flow looks like this:
-
Event creation: An event is defined and emitted using the
.emit()
method. -
Listener registration: Listeners are registered to an event using the
.on()
method. -
Event handling: Once the event is emitted, the registered listeners are triggered and execute their respective callbacks.
Basic Example of EventEmitter
Let’s start with a basic example. First, you’ll need to import the EventEmitter
from Node.js’s events
module and instantiate it.
const EventEmitter = require('events);
const eventEmitter = new EventEmitter()
//define an event listner
eventEmitter.on('great', (name) => {
console.log(`hello , ${name}!`);
})
//emit the greet event
eventEmitter.emit('greet', 'Steve');
In the code above :
-
We create an instance of
EventEmitter
. -
We set up an event listener using
.on()
for the event named'greet'
. This listener takes a callback function that logs a greeting to the console. -
We trigger (emit) the event using
.emit()
. When the event is emitted, it passes'Steve'
as an argument to the listener, resulting in the output:
hello
Key Methods of an EventEmitter
1. .on(event, listener)
The .on()
method registers an event listener for the specified event. Every time the event is emitted, the listener callback function is executed.
eventEmitter
2. .emit(event, [arg1], [arg2], [...])
The .emit()
method triggers an event, optionally passing arguments to the event listener callbacks.
eventEmitter
3. .once(event, listener)
The .once()
method works like .on()
, but the listener is called only the first time the event is emitted. After the event is handled once, the listener is automatically removed.
eventEmitter
4. .off(event, listener)
or .removeListener(event, listener)
The .off()
or .removeListener()
method removes a previously registered event listener.
const greetListener = (name) => {
console.log(`Hello, ${name}!`);
};
eventEmitter.on('greet', greetListener);
// Remove the event listener
eventEmitter.off('greet', greetListener);
eventEmitter.emit('greet', 'Steve'); // No output, listener has been removed
5. .removeAllListeners([event])
This method removes all listeners for a given event. If no event is specified, it removes all listeners for all events.
eventEmitter
Practical Use Case: File Reading with EventEmitter
In real-world applications, you often use the EventEmitter
to handle asynchronous events. Let’s simulate a scenario where you read a file, and once the file is read, an event is emitted to notify other parts of the application.
const EventEmitter = require('events');
const fs = require('fs');
const eventEmitter = new EventEmitter();
// Register listener for the 'fileRead' event
eventEmitter.on('fileRead', (content) => {
console.log('File content:', content);
});
// Asynchronously read a file and emit event when done
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
} else {
eventEmitter.emit('fileRead', data); // Emit event with file data
}
});
In this example:
-
We listen to the
fileRead
event. -
When the file is successfully read, we emit the
fileRead
event and pass the file's content to the event listener. -
The event listener logs the file content when the event is triggered.
This pattern is useful in many situations, like when handling database connections, HTTP requests, or any time-consuming operations.
Working with Multiple Event Listeners
You can register multiple listeners for the same event. Each listener will be executed in the order they were registered.
javascripteventEmitter
Output:
First listener: Hello, Steve! Second listener: Hi there, Steve!
In this example, both listeners of the
greet
event is executed when the event is emitted.EventEmitter in Real-Time Systems
Node.js uses the
EventEmitter
pattern extensively under the hood, especially for real-time systems like web servers and networking applications. A good example is HTTP servers in Node.js.Here’s how the
EventEmitter
pattern is used in a basic HTTP server:const http = require('http'); const server = http.createServer((req, res) => { if (req.url === '/') { res.write('Welcome to the homepage!'); res.end(); } }); server.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, the
server
object is an instance ofEventEmitter
. When a request is received, an event ('request'
) is emitted, and the provided callback is executed.Custom Error Handling with EventEmitter
Handling errors effectively is a crucial aspect of building robust applications. With this, you can emit and listen for error events.
const EventEmitter = require('events'); const eventEmitter = new EventEmitter(); // Register a listener for error events eventEmitter.on('error', (err) => { console.error('Error occurred:', err.message); }); // Emit an error event eventEmitter.emit('error', new Error('Something went wrong!'));
This pattern is commonly used in scenarios where an error occurs asynchronously, allowing other parts of your application to respond to the error gracefully.
The Event Loop and EventEmitter
One of the reasons Node.js is efficient and scalable is its event loop. The
EventEmitter
operates within this event loop, enabling it to handle asynchronous events without blocking the main execution thread.When an event is emitted, Node.js checks the event loop and executes any registered listeners. The event loop allows Node.js to handle thousands of concurrent events without blocking, making it ideal for I/O-bound tasks like reading from databases or interacting with files.
Removing Listeners
Over time, you may need to clean up listeners, especially if you are dealing with a long-running application where listeners are created and destroyed dynamically.
-
Remove a specific listener: As shown earlier, you can use
.off()
or.removeListener()
to remove specific listeners. -
Remove all listeners for a specific event: Use
.removeAllListeners()
to clear all listeners tied to a specific event. -
Remove all listeners for all events: Call
.removeAllListeners()
without any arguments.
Memory Leaks and MaxListeners
If you add too many listeners for the same event, you might encounter a memory leak warning in Node.js:
(node:12345) MaxListenersExceededWarning: Possible EventEmitter memory leak detected. 11 listeners added.
By default, Node.js allows up to 10 listeners per event. If you need more, you can increase the limit using the
setMaxListeners()
method:javascripteventEmitter
However, be cautious—having too many listeners could indicate a potential memory leak in your application.
Explaining EventEmitter to a 5-Year-Old
Imagine you’re throwing a party, and you have a whistle. You tell your friends, "Whenever I blow the whistle, everyone shouts 'Hooray!'" Now, when you blow the whistle (emit an event), your friends (the listeners) will all shout "Hooray!" (execute their callbacks). If a new friend arrives at the party and you tell them the same thing, they’ll also shout when the whistle blows. This is how
EventEmitter
works.Conclusion
The
EventEmitter
class is a vital tool in the Node.js ecosystem, enabling an efficient event-driven architecture. By emitting and listening to events, you can handle asynchronous tasks smoothly, from file operations to complex real-time systems. Understanding how to leverageEventEmitter
allows you to write cleaner, more modular, and more efficient Node.js applications. -